useDebugValue
useDebugValue
is a React Hook that lets you add a label to a custom Hook in React DevTools.
useDebugValue(value, format?)
Reference
useDebugValue(value, format?)
Call useDebugValue
at the top level of your custom Hook to display a readable debug value:
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
Parameters
value
: The value you want to display in React DevTools. It can have any type.- optional
format
: A formatting function. When the component is inspected, React DevTools will call the formatting function with thevalue
as the argument, and then display the returned formatted value (which may have any type). If you don’t specify the formatting function, the originalvalue
itself will be displayed.
Returns
useDebugValue
does not return anything.
Usage
Adding a label to a custom Hook
Call useDebugValue
at the top level of your custom Hook to display a readable debug value for React DevTools.
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
This gives components calling useOnlineStatus
a label like OnlineStatus: "Online"
when you inspect them:
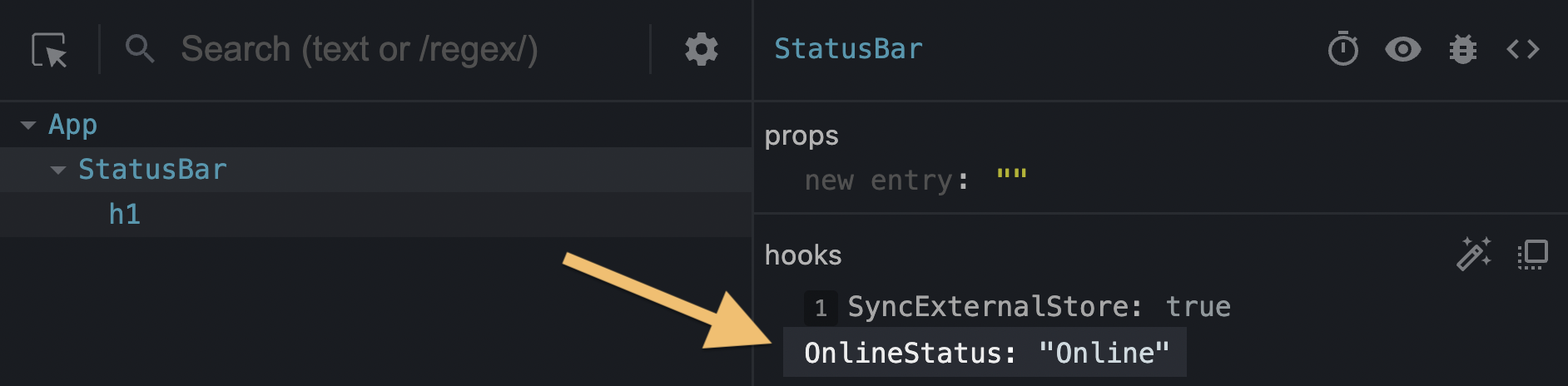
Without the useDebugValue
call, only the underlying data (in this example, true
) would be displayed.
import { useSyncExternalStore, useDebugValue } from 'react'; export function useOnlineStatus() { const isOnline = useSyncExternalStore(subscribe, () => navigator.onLine, () => true); useDebugValue(isOnline ? 'Online' : 'Offline'); return isOnline; } function subscribe(callback) { window.addEventListener('online', callback); window.addEventListener('offline', callback); return () => { window.removeEventListener('online', callback); window.removeEventListener('offline', callback); }; }
Deferring formatting of a debug value
You can also pass a formatting function as the second argument to useDebugValue
:
useDebugValue(date, date => date.toDateString());
Your formatting function will receive the debug value as a parameter and should return a formatted display value. When your component is inspected, React DevTools will call this function and display its result.
This lets you avoid running potentially expensive formatting logic unless the component is actually inspected. For example, if date
is a Date value, this avoids calling toDateString()
on it for every render.